Debunking Python Performance Myths for MATLAB Users
A fair comparison of Python's vs MATLAB's performance, debunking common myths with benchmarks and evidence to help you make informed migration decisions.
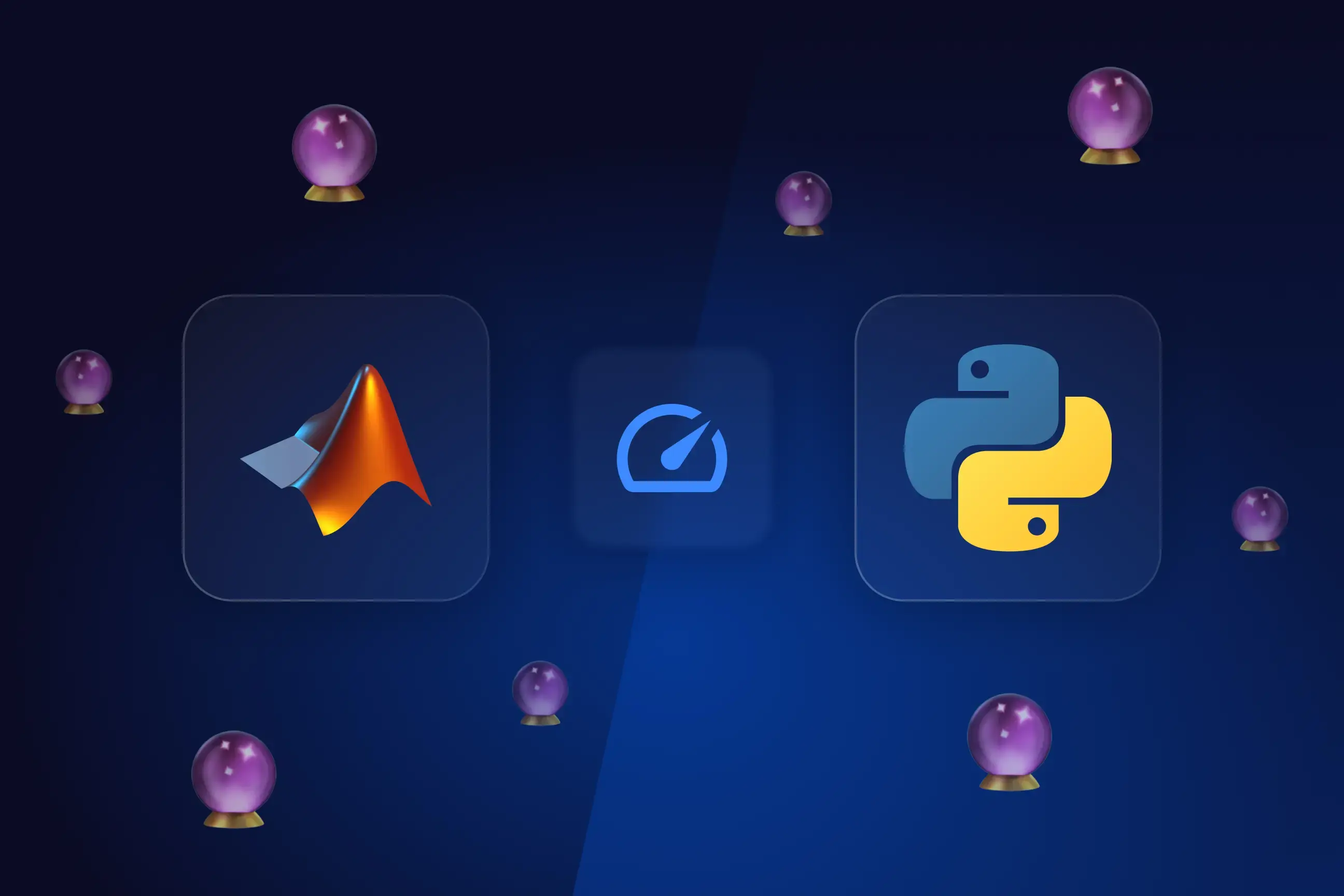
Recently, I posted an article on LinkedIn about the growing trend of MATLAB users migrating to Python. As expected, many loyal MATLAB users weren’t particularly convinced—which is fine, there's still a lot to love about MATLAB, However, I was irked by some beliefs about Python’s performance that I felt were woefully out-of-date or just plain incorrect, Thus, here’s a follow-up article to debunk some of the most common myths about Python that I’ve seen in the wild.
Before I start, I hope that I made it clear in the original article that I was not comparing pure Python to vanilla MATLAB. Rather, pure Python and its ecosystem of libraries for matrix operations and scientific computing. Likewise, my comparison also encompasses MATLAB’s ecosystem of toolboxes such as Simulink and Symbolic Math.
With that in mind, let’s get into the list.
Myth 1: "MATLAB’s matrix operations are unbeatable"
MATLAB advocates often point out that MATLAB was literally built for matrix operations - it's right there in the name! Nothing can come close. Indeed, MATLAB used to be superior to anything in the Python ecosystem, but thanks to recent optimizations, the difference is now negligible.
NumPy’s memory management has improved
Where NumPy really pulls ahead is in how it manages memory, and this has a huge impact on performance in real-world applications. One of its biggest advantages is views—when you slice an array in NumPy, you’re often just creating a new “window” into the same memory rather than copying the data.
This means operations on subarrays happen instantly and without extra memory overhead. MATLAB, on the other hand, tends to create copies when slicing, meaning if you extract a column from a large matrix, MATLAB will usually allocate a whole new array for it. That might not sound like much, but when you’re dealing with large datasets, those extra copies add up fast, burning through both memory and CPU cycles.
NumPy also lets you choose your data type explicitly. If you don’t need full double precision (float64), you can store numbers in float32, int16, or whatever best fits your needs—cutting memory usage in half or more. MATLAB, by default, stores everything as float64, so unless you explicitly convert data types, you’re always using more memory than necessary. This can matter a lot when dealing with massive arrays.
NumPy is also good at working with datasets larger than RAM. It has built-in support for memory mapping, meaning you can load just a chunk of a file into memory instead of the whole thing. MATLAB has something similar (matfile), but it’s more restrictive and doesn’t integrate as seamlessly into workflows where you need direct, efficient access to huge datasets.
Now, to be fair, MATLAB still has an edge in some areas. Its sparse matrix operations are well-optimized, and some built-in functions are faster because MATLAB has fine-tuned them over decades. But NumPy makes up for that with array broadcasting, stride-based memory access, and direct integration with SciPy, which covers most use cases just as well.
Thus, for most real-world applications, neither has a significant speed advantage over the other—it really comes down to ecosystem and workflow.
CuPy is even faster than NumPy
GPU acceleration has been a real game-changer for Python’s performance on matrix operations. Libraries like CuPy enable NumPy-like syntax on GPUs, allowing you to achieve dramatic speedups over CPU-bound operations. For example, Unum, a Deep-Tech research company, benchmarked CuPy against vanilla NumPy (using MKL—the fastest CPU backend and the same one that MATLAB uses). Granted the GPU didn't make everything faster (frequently moving data on and off the GPU can slow things down) but they found that CuPy sped up many operations significantly. Here's a sample:
- GEMM (General Matrix Multiplication): Speedups range from 8.3x up to 25.1x1.
- Moving Average: Speedups range from 10.9x to 59.4x1.
- Medians: Speedups range from 11.2x to 99.4x1.
- Sorting: Speedups range from 87.7x to 1008.5x1 (sorting big arrays is one of the biggest strengths of GPUs)
Because the comparison is using MKL, these speedups apply to CPU-bound MATLAB too.
Of course MATLAB also supports GPU operations, but it comes with additional licensing costs and restrictions. You’ll need to shell out for the Parallel Computing Toolbox and for every worker that uses a CPU core and/or GPU. Since the equivalent Python tools are mostly open source, the workers are free. Unlike MATLAB, your capacity to scale is far less constrained by your budget. Of course, you’ll still have to pay something for cloud compute costs such as GPU and CPU hours, but you’ll be paying for that no matter what framework you use.
Myth 2: "Python’s interpreted nature inherently makes it slower"
While this might have been a valid concern in the early days of scientific Python, it's simply not the case anymore.
The reasons are simple:
Scientific Python usually leverages compiled backends
When you're doing serious computational work, you're using libraries like NumPy and SciPy, which are essentially thin Python wrappers around highly optimized C and Fortran code. These libraries are often using the exact same underlying BLAS (Basic Linear Algebra Subprograms) and LAPACK (Linear Algebra PACKage) routines that MATLAB uses.
However, I will concede that there are some important details that can affect performance.
Among the many BLAS implementations, NumPy generally uses OpenBLAS by default, whereas MATLAB uses the BLAS implementation from Intel’s MKL (Math Kernel Library). This BLAS flavor is optimized for Intel CPUs and can be a lot faster than OpenBLAS for some tasks (not so much on AMD CPUs). You can of course configure Numpy to use MKL for optimized BLAS and LAPACK operations instead. Also, Anaconda distributes an MKL-optimized build of NumPy, so you get MKL by default in conda environments anyway.
Aside from the BLAS flavor, there are many other variables that can affect speed such as the size of matrices (with there being little difference between Numpy and MATLAB on matrices larger than 2000 dimensions), but I don’t want to get too deep into the weeds on BLAS and LAPACK here.
My main point is that performance is largely dependent on the underlying mathematical kernels rather than Python's inherent slowness as an interpreted language.
Beyond BLAS and LAPACK, vectorization gives NumPy another key performance advantage.
NumPy’s vectorization bypasses the interpreter
MATLAB’s speed advantage traditionally comes from Just-in-Time (JIT) compilation, which optimizes loops and function calls dynamically at runtime. In contrast, NumPy's performance primarily comes from vectorization—the process of applying operations to entire arrays and matrices at once, rather than using explicit loops.
Under the hood, NumPy executes this as a single, optimized batch operation in compiled code, avoiding the overhead of Python loops.
For example, instead of writing a loop to add two arrays element by element (slow in Python):
result = []
for i in range(len(array1)):
result.append(array1[i] + array2[i])
You simply write:
result = array1 + array2
The vectorized version delegates the entire operation to NumPy's compiled C code, bypassing Python's interpreter overhead completely. This means that for most numerical workloads, NumPy is already competitive with MATLAB without requiring JIT acceleration.
Thus, it’s a common misconception that Python is inherently slow for scientific computing because it’s an interpreted language. The reality is that most performance-critical computations in Python are offloaded to compiled, optimized code—whether through vectorized NumPy operations or optimized BLAS and LAPACK routines. The interpretation overhead of Python itself is pretty much negligible in real-world applications.
Myth 3: "Loops Are Always Faster in MATLAB"
This one is particularly sticky because it used to be true, and old beliefs die hard in the software world. But, as always, things change fast. In post-2018 versions of MATLAB, the JIT compiler accelerated loops dramatically, making Python’s loops look irredeemably slow in comparison. Nowadays, Python now has Numba— its own JIT compiler, which makes running nested loops just as fast.
Numba can make Python loops run faster than MATLAB

The secret to Numba’s speed lies in how it approaches optimization. Unlike MATLAB's JIT compiler, which basically has one strategy for loop optimization, Numba can analyze your loop patterns and generate machine code that's specifically optimized for your particular use case. Thus, the claim that loops are faster in MATLAB is very outdated.
For example, John T. Foster, Jr, an associate professor at the The University of Texas proved this in an excellent rebuttal to a rather aggressive takedown of Python on the Mathworks website (The Mathworks attitude to towards Python has since been toned down, you can find of the original on the Wayback Machine).
Foster used an example comparing the performance of adding one to a million numbers in both Python and MATLAB, with various optimization techniques. The vanilla Python implementation used a for loop to iterate through an array and add one to each element.
The pure Python approach was predictably slow, but with optimizations it outperformed MATLAB. More specifically, he found the fastest Python implementation (NumPy + Numba JIT with parallel execution) outperformed out the fastest MATLAB implementation which used vectorization.
Myth 4: "Python Sacrifices Numerical Accuracy for Speed"
This is perhaps the most surprising misconception I encounter: the idea that Python somehow sacrifices numerical accuracy for speed. This myth probably stems from Python's dynamic typing system, but it's completely unfounded when it comes to scientific computing.
The reality is that both Python's NumPy and MATLAB use IEEE 754 double-precision floating-point arithmetic by default. The difference in the numerical precision of basic operations between the two platforms is minimal. In fact, Python often provides more flexibility in terms of numerical precision.
What's particularly interesting is that Python's scientific stack often provides more control over numerical precision than vanilla MATLAB. Modules like mpmath and decimal allow for arbitrary-precision arithmetic when needed, something that's not easily available in MATLAB without additional toolboxes such as the MATLAB’s Symbolic Math Toolbox.
FACT: MATLAB beats Python when you need “hard real-time” performance
In the interest of fairness, I want to highlight one area where MATLAB does hold a real edge over Python: Hardware-in-the-Loop (HIL) testing. HIL testing in automotive and aerospace requires hard real-time performance, often under 10 microseconds of jitter. When validating an ECU or flight control system, even tiny delays can cause failures. You need predictable, deterministic behavior. MATLAB and Simulink Real-Time can fulfill this requirement but only when paired with specialized hardware such as Speedgoat. Python, on the other hand, will never be fast enough, no matter how beefy your hardware is.
Python’s architecture makes it difficult to get precise, deterministic behavior—its Global Interpreter Lock (GIL) and garbage collection introduce unpredictable pauses, sometimes up to 15 milliseconds, which makes it unsuitable for strict real-time applications.
MATLAB’s toolchain achieves 250-nanosecond timestamp accuracy, while Python struggles to go below 50 microseconds with TCP/IP. Simulink’s auto-generated C code bypasses interpretation, enabling control loops over 100 kHz.
That said, Python still has a place in HIL. Many organizations use it for test scenario generation, data analysis, and visualization, while MATLAB handles the real-time execution. For soft real-time needs (where occasional timing variations of up to 10 milliseconds are acceptable), there are several Python frameworks that can do the job. But for microsecond-level precision, MATLAB/Simulink with Speedgoat remains the gold standard—which is fitting, since you’ll need a small fortune in gold to afford it.
The Bigger Picture
Let's be frank about what's really at stake here: budgets. While both platforms can deliver excellent performance, clinging to MATLAB solely because of these outdated misconceptions is going to cost you.
A MATLAB license with the necessary toolboxes can easily cost tens of thousands of dollars per seat annually. For a medium-sized engineering team, this can translate to hundreds of thousands of dollars in licensing fees alone.
This isn't just about saving on license fees. Python's broader ecosystem and integration capabilities often mean faster development cycles, easier deployment, and more efficient collaboration. When you factor in these operational efficiencies along with the licensing costs, the financial impact of staying with MATLAB due to misconceptions could be costing your organization six or seven figures annually.
Unless you have a specific, irreplaceable MATLAB toolbox that's crucial to your work - or you truly need hard real-time HIL capabilities with microsecond-level determinism - these performance myths shouldn't be the reason you're paying those premiums. The Python ecosystem has matured to the point where it's not just a viable alternative - it's often the more capable platform.
Making the switch requires some investment in training and transition, certainly. But the long-term financial benefits make this a compelling business decision, not just a technical one.
What’s a Rich Text element?
The rich text element allows you to create and format headings, paragraphs, blockquotes, images, and video all in one place instead of having to add and format them individually. Just double-click and easily create content.
Static and dynamic content editing
A rich text element can be used with static or dynamic content. For static content, just drop it into any page and begin editing. For dynamic content, add a rich text field to any collection and then connect a rich text element to that field in the settings panel. Voila!
How to customize formatting for each rich text
Headings, paragraphs, blockquotes, figures, images, and figure captions can all be styled after a class is added to the rich text element using the "When inside of" nested selector system.
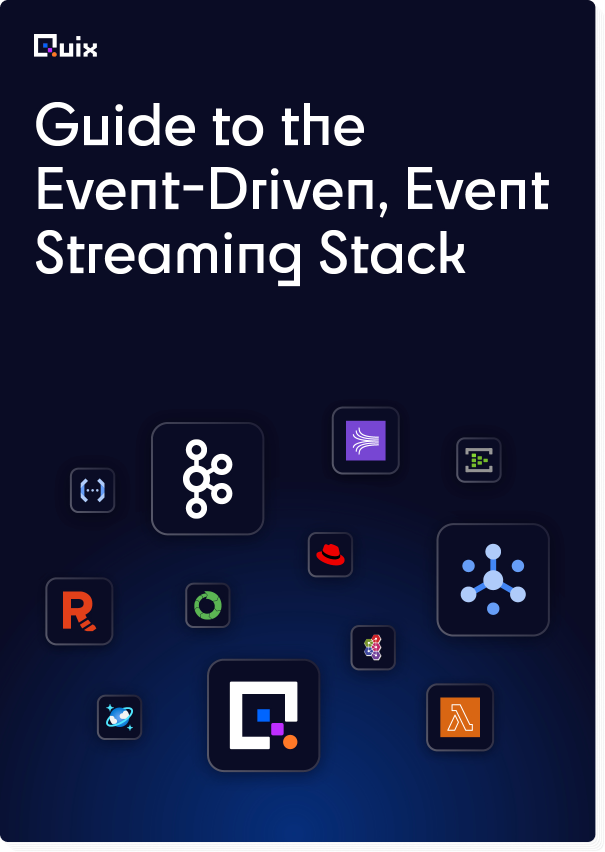
Check out the repo
Our Python client library is open source, and brings DataFrames and the Python ecosystem to stream processing.

Interested in Quix Cloud?
Take a look around and explore the features of our platform.

Interested in Quix Cloud?
Take a look around and explore the features of our platform.
